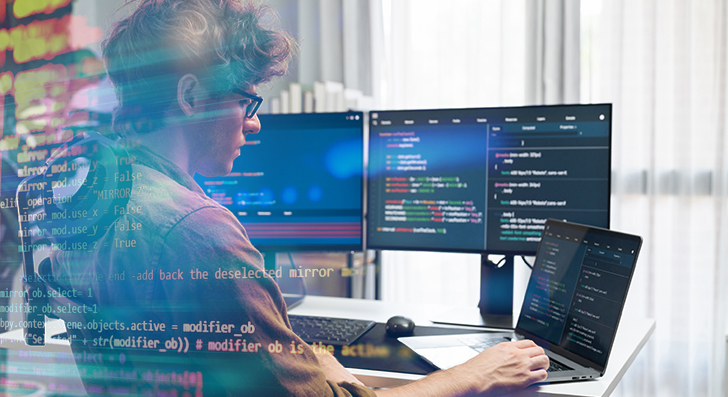
Scalability means your software can take care of development—more buyers, additional info, and even more site visitors—without the need of breaking. For a developer, creating with scalability in mind will save time and anxiety afterwards. Below’s a clear and simple guideline that may help you commence by Gustavo Woltmann.
Design for Scalability from the beginning
Scalability isn't a thing you bolt on later—it ought to be component of your system from the beginning. Quite a few applications fall short once they improve quickly for the reason that the initial design and style can’t tackle the extra load. For a developer, you have to Assume early about how your technique will behave stressed.
Begin by designing your architecture to get flexible. Stay away from monolithic codebases where by every thing is tightly linked. In its place, use modular design and style or microservices. These designs crack your app into lesser, independent elements. Just about every module or services can scale on its own devoid of affecting the whole process.
Also, contemplate your database from day one particular. Will it have to have to handle one million customers or maybe 100? Select the suitable type—relational or NoSQL—depending on how your facts will mature. Plan for sharding, indexing, and backups early, even if you don’t want them nevertheless.
A different crucial position is to stop hardcoding assumptions. Don’t generate code that only works below existing problems. Contemplate what would happen if your consumer base doubled tomorrow. Would your app crash? Would the database slow down?
Use style patterns that assistance scaling, like message queues or occasion-driven programs. These enable your application tackle extra requests without the need of finding overloaded.
Any time you Make with scalability in mind, you're not just planning for achievement—you are decreasing future problems. A perfectly-prepared technique is simpler to maintain, adapt, and mature. It’s much better to prepare early than to rebuild afterwards.
Use the appropriate Database
Selecting the right databases can be a crucial part of developing scalable applications. Not all databases are designed precisely the same, and using the Completely wrong one can slow you down or simply bring about failures as your app grows.
Start by comprehending your data. Could it be extremely structured, like rows inside of a desk? If Sure, a relational databases like PostgreSQL or MySQL is an effective fit. These are definitely sturdy with associations, transactions, and consistency. In addition they assistance scaling procedures like read through replicas, indexing, and partitioning to handle additional visitors and details.
When your data is a lot more flexible—like consumer activity logs, merchandise catalogs, or files—take into account a NoSQL option like MongoDB, Cassandra, or DynamoDB. NoSQL databases are greater at managing huge volumes of unstructured or semi-structured details and might scale horizontally more simply.
Also, consider your read through and write designs. Are you presently carrying out many reads with fewer writes? Use caching and browse replicas. Are you currently dealing with a major create load? Investigate databases which can deal with substantial generate throughput, or perhaps function-dependent details storage programs like Apache Kafka (for non permanent data streams).
It’s also wise to Consider in advance. You might not require Superior scaling characteristics now, but deciding on a databases that supports them implies you gained’t will need to modify afterwards.
Use indexing to hurry up queries. Avoid pointless joins. Normalize or denormalize your information dependant upon your entry styles. And generally keep track of database functionality while you increase.
Briefly, the appropriate databases will depend on your application’s framework, pace wants, And exactly how you be expecting it to improve. Acquire time to choose correctly—it’ll preserve plenty of problems later.
Optimize Code and Queries
Speedy code is essential to scalability. As your application grows, each individual smaller hold off adds up. Poorly written code or unoptimized queries can decelerate effectiveness and overload your technique. That’s why it’s crucial that you Construct effective logic from the beginning.
Begin by writing clean up, basic code. Stay away from repeating logic and remove just about anything unwanted. Don’t select the most complex Alternative if an easy 1 operates. Keep your features quick, concentrated, and simple to test. Use profiling applications to seek out bottlenecks—locations where your code can take way too lengthy to run or takes advantage of excessive memory.
Up coming, evaluate your database queries. These often sluggish issues down much more than the code itself. Be certain Each and every question only asks for the info you actually will need. Stay away from Find *, which fetches every little thing, and in its place pick unique fields. Use indexes to speed up lookups. And stay clear of carrying out a lot of joins, Particularly throughout big tables.
When you discover the exact same information currently being asked for repeatedly, use caching. Keep the results briefly working with tools like Redis or Memcached and that means you don’t need to repeat high-priced functions.
Also, batch your databases operations once you can. In place of updating a row one after the other, update them in groups. This cuts down on overhead and helps make your app additional economical.
Remember to check with significant datasets. Code and queries that work fantastic with one hundred data could crash every time they have to handle 1 million.
In brief, scalable apps are rapidly applications. Keep the code tight, your queries lean, and use caching when desired. These steps aid your application remain clean and responsive, at the same time as the load boosts.
Leverage Load Balancing and Caching
As your application grows, it's got to take care of more consumers and a lot more website traffic. If every thing goes by means of one particular server, it can promptly become a bottleneck. That’s exactly where load balancing and caching are available in. These two equipment enable keep the application speedy, stable, and scalable.
Load balancing spreads incoming site visitors throughout multiple servers. Rather than 1 server carrying out the many get the job done, the load balancer routes buyers to various servers depending on availability. This implies no single server gets overloaded. If a person server goes down, the load balancer can send out traffic to the others. Applications like Nginx, HAProxy, or cloud-primarily based solutions from AWS and Google Cloud make this straightforward to arrange.
Caching is about storing facts briefly so it may be reused rapidly. When customers ask for the same information and facts all over again—like an item web site or simply a profile—you don’t should fetch it with the database each and every time. You are able to provide it through the cache.
There are two common types of caching:
1. Server-facet caching (like Redis or Memcached) suppliers knowledge in memory for rapid accessibility.
two. Consumer-facet caching (like browser caching or CDN caching) outlets static files near to the user.
Caching cuts down database load, increases speed, and makes your application much more successful.
Use caching for website things which don’t alter often. And constantly ensure your cache is updated when knowledge does change.
To put it briefly, load balancing and caching are easy but highly effective resources. Alongside one another, they help your app tackle a lot more consumers, continue to be quick, and Get better from troubles. If you propose to develop, you may need both.
Use Cloud and Container Equipment
To develop scalable applications, you will need instruments that permit your app expand quickly. That’s the place cloud platforms and containers can be found in. They offer you flexibility, lessen set up time, and make scaling much smoother.
Cloud platforms like Amazon Internet Companies (AWS), Google Cloud System (GCP), and Microsoft Azure Enable you to hire servers and services as you will need them. You don’t really need to obtain components or guess future capability. When targeted visitors increases, it is possible to insert additional means with just some clicks or automatically utilizing auto-scaling. When visitors drops, you are able to scale down to save money.
These platforms also supply companies like managed databases, storage, load balancing, and protection instruments. You may center on building your application instead of running infrastructure.
Containers are A further vital Software. A container deals your app and every thing it must operate—code, libraries, configurations—into one particular unit. This makes it easy to maneuver your application among environments, from the laptop towards the cloud, without surprises. Docker is the most popular Software for this.
Once your app utilizes multiple containers, instruments like Kubernetes allow you to control them. Kubernetes handles deployment, scaling, and recovery. If just one element of one's application crashes, it restarts it routinely.
Containers also ensure it is easy to separate aspects of your app into services. You may update or scale areas independently, that is perfect for efficiency and reliability.
Briefly, utilizing cloud and container applications implies you can scale rapidly, deploy effortlessly, and Get better swiftly when problems come about. If you want your app to improve with out boundaries, begin employing these tools early. They help save time, reduce possibility, and enable you to continue to be focused on making, not fixing.
Watch Every thing
When you don’t monitor your application, you received’t know when issues go Completely wrong. Monitoring will help the thing is how your application is carrying out, place challenges early, and make better choices as your application grows. It’s a critical A part of constructing scalable units.
Start by tracking standard metrics like CPU utilization, memory, disk House, and response time. These tell you how your servers and providers are executing. Resources like Prometheus, Grafana, Datadog, or New Relic can assist you accumulate and visualize this details.
Don’t just monitor your servers—keep track of your app far too. Regulate how much time it takes for customers to load webpages, how often mistakes come about, and the place they come about. Logging applications like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will let you see what’s going on within your code.
Arrange alerts for important problems. For instance, When your response time goes previously mentioned a limit or even a support goes down, you must get notified quickly. This will help you deal with troubles rapidly, typically ahead of consumers even recognize.
Monitoring can also be helpful whenever you make changes. If you deploy a new aspect and find out a spike in problems or slowdowns, you could roll it back again ahead of it triggers real damage.
As your application grows, targeted traffic and information increase. Devoid of checking, you’ll skip indications of difficulties until finally it’s too late. But with the ideal equipment in place, you keep in control.
To put it briefly, monitoring helps you maintain your app trusted and scalable. It’s not nearly spotting failures—it’s about being familiar with your technique and making sure it works well, even under pressure.
Remaining Feelings
Scalability isn’t only for huge companies. Even modest applications need to have a strong foundation. By building very carefully, optimizing sensibly, and using the ideal resources, you could Construct applications that grow efficiently without breaking under pressure. Start out small, think huge, and Establish intelligent.